View the whole notebook: https://github.com/mattclifford1/IQM-Vis/tree/main/dev_resources/docs/notebooks/Tutorial_2-Customisation.ipynb
Tutorial 2: Simple Customisation
In this notebook we will get to grips with how to peice together a simple IQM-Vis UI. We will go through the 3 basic aspects of customisation: - Images - Metrics - Transformations
Let’s start by importing the package.
[ ]:
import IQM_Vis
Images
To include custom images we define their filepaths. For now we will use the example images provided with IQM-Vis, but feel free to change the file paths with your own local image files.
[2]:
image1 = IQM_Vis.examples.images.IMAGE1
image2 = IQM_Vis.examples.images.IMAGE2
images = [image1, image2]
print(f'Images files: {images}')
Images files: ['/home/matt/projects/IQM-VIS/IQM_Vis/examples/images/waves1.jpeg', '/home/matt/projects/IQM-VIS/IQM_Vis/examples/images/waves2.jpeg']
Metrics
To include some custom image quality metrics we store them in a dictionary. These return a scalar value when comparing two images. For now we will use some metrics provided by IQM-Vis. For a list of all metrics provided by IQM-Vis see the documentation (https://mattclifford1.github.io/IQM-Vis/IQM_Vis.metrics.html). See later tutorials for how to define your own custom metrics.
[3]:
MAE = IQM_Vis.IQMs.MAE()
MSE = IQM_Vis.IQMs.MSE()
SSIM = IQM_Vis.IQMs.SSIM()
metrics = {'MAE': MAE,
'MSE': MSE,
'1-SSIM': SSIM}
Metric Images
Adding image quality metrics that return an image instead of a scalar value is a similar process to the metrics before. Defining these is optional but can be useful for qualitive spacial analysis.
[4]:
MSE_image = IQM_Vis.IQMs.MSE(return_image=True)
SSIM_image = IQM_Vis.IQMs.SSIM(return_image=True)
metric_images = {'MSE': MSE_image,
'1-SSIM': SSIM_image}
Transformations
Defining image transformation/distortions requires a little more information as we also need to provide the range of values that we want the transformations to operate over.
[5]:
rotation = IQM_Vis.transforms.rotation
blur = IQM_Vis.transforms.blur
brightness = IQM_Vis.transforms.brightness
jpeg_compression = IQM_Vis.transforms.jpeg_compression
transformations = {
'rotation': {'min':-180, 'max':180, 'function':rotation}, # normal input
'blur': {'min':1, 'max':41, 'function':blur, 'normalise':'odd'}, # only odd ints since it's a kernel
'brightness':{'min':-1.0, 'max':1.0, 'function':brightness}, # float values
'jpg comp.': {'min':1, 'max':100, 'function':jpeg_compression, 'init_value':100}, # non zero inital value
}
Putting it all together
We need to pass everything to the UI maker
[ ]:
IQM_Vis.make_UI(transformations=transformations,
image_list=images,
metrics=metrics,
metric_images=metric_images)
You will now get the desired UI with the specified transformations, metrics and images
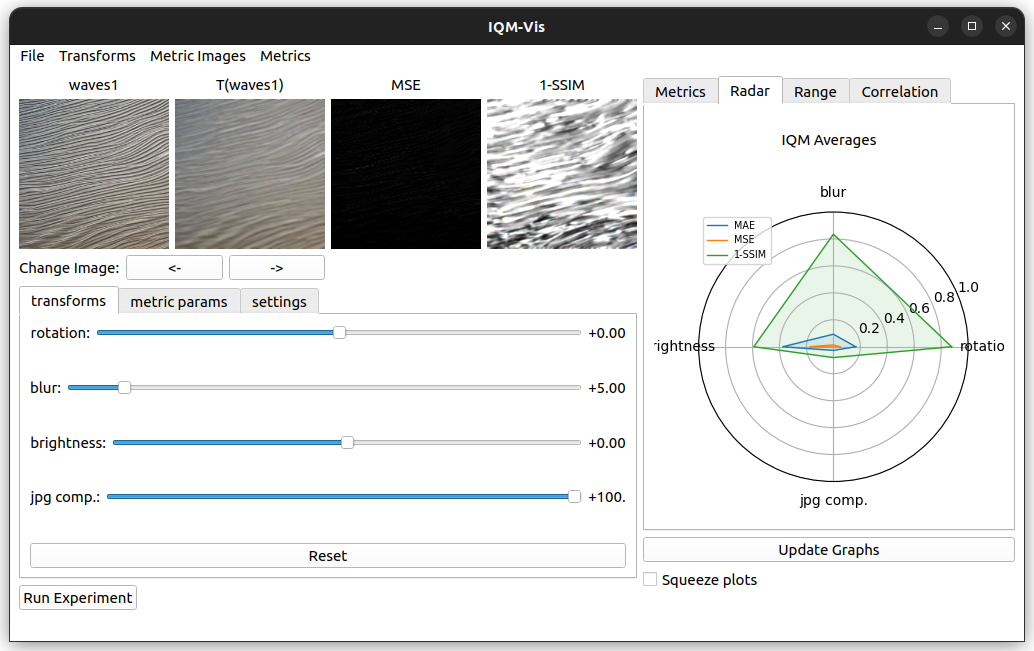